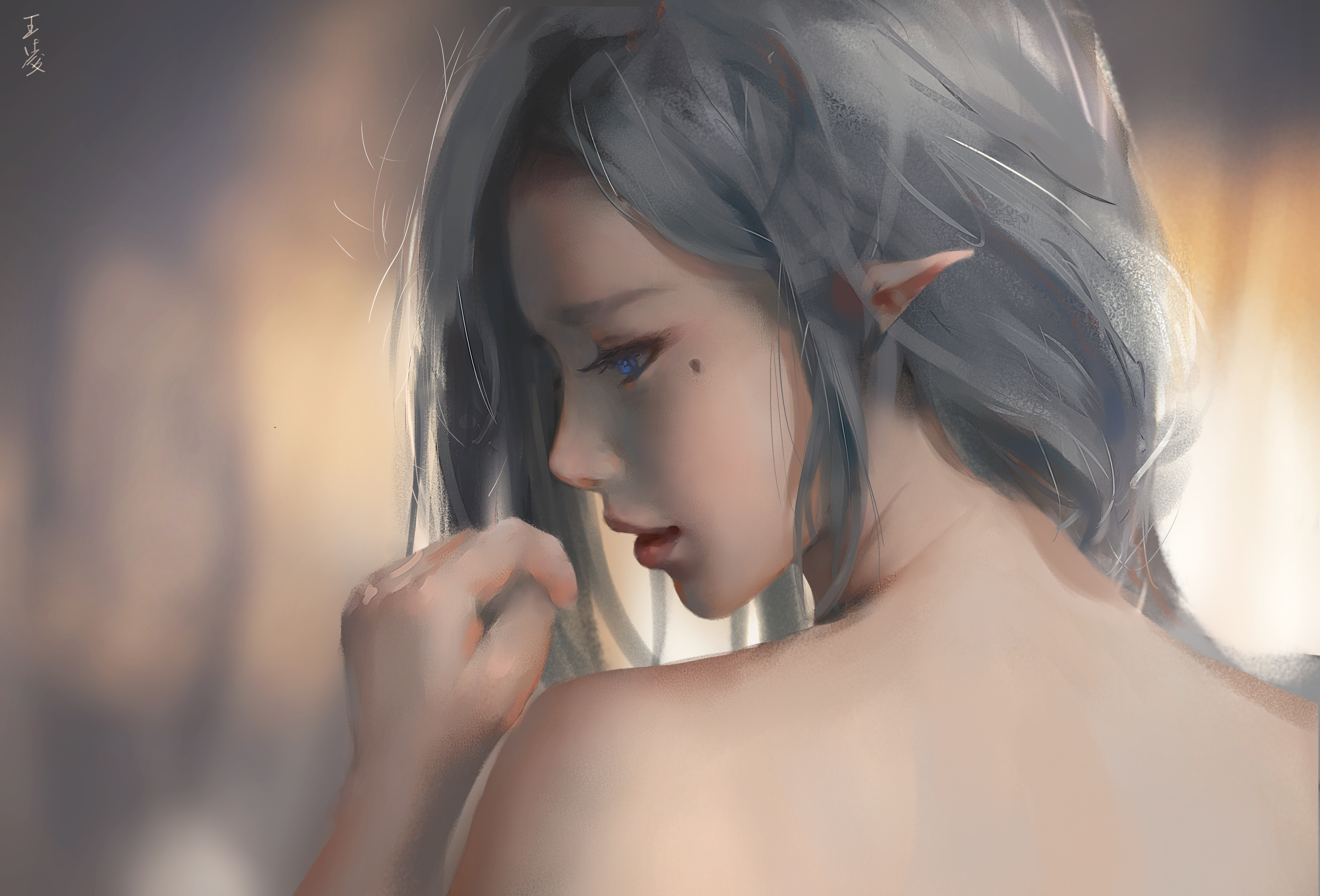
反射
反射机制是在运行状态中
对于任意一个类、都能够知道这个类所有的属性和方法
对于任意一个对象、都能够调用他的任意一个属性和方法
反射提供的功能
运行时判断任意一个对象所属的类
运行时构造任意一个类的对象
运行时判断任意一个类所具有的成员变量和方法
运行时调用一个对象的方法
生成动态代理
反射入口
获取Class的三种方法
1 2 3 4 5 6 7
| Class<?> clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); System.out.println(clazz); } catch (ClassNotFoundException e) { e.printStackTrace(); }
|
1
| System.out.println(MyInterfaceImpl.class);
|
1 2 3
| MyInterfaceImpl myInterface = new MyInterfaceImpl(); Class<? extends MyInterfaceImpl> aClass = myInterface.getClass(); System.out.println(aClass);
|
获取方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| Class<?> clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); Method[] methods = clazz.getMethods(); for(Method method: methods){ System.out.println(method); } System.out.println("-------------"); Method[] declaredMethods = clazz.getDeclaredMethods(); for (Method declare:declaredMethods){ System.out.println(declare); } } catch (ClassNotFoundException e) { e.printStackTrace(); }
|
公共方法:本类以及父类、接口中的所有方法
符合的访问修饰符规律的方法
获取接口
1 2 3 4 5 6 7 8 9 10
| Class clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); Class[] interfaces = clazz.getInterfaces(); for (Class inter: interfaces){ System.out.println(inter); } } catch (ClassNotFoundException e) { e.printStackTrace(); }
|
获取父类
1 2 3 4 5 6 7 8
| Class clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); Class superclass = clazz.getSuperclass(); System.out.println(superclass); } catch (ClassNotFoundException e) { e.printStackTrace(); }
|
获取构造方法
1 2 3 4 5 6 7 8 9 10
| Class clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); Constructor[] constructors = clazz.getConstructors(); for(Constructor constructor: constructors){ System.out.println(constructor); } } catch (ClassNotFoundException e) { e.printStackTrace(); }
|
获取属性
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| Class clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); Field[] fields = clazz.getFields(); for (Field field : fields){ System.out.println(field); } Field[] declaredFields = clazz.getDeclaredFields(); for (Field fie : declaredFields){ System.out.println(fie); } } catch (ClassNotFoundException e) { e.printStackTrace(); }
|
获取类的类的对象
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15
| Class clazz = null; try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); MyInterfaceImpl o = (MyInterfaceImpl)clazz.newInstance(); o.getInterface1(); o.getInterface2();
}catch (ClassNotFoundException e){ e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (InstantiationException e) { e.printStackTrace(); }
|
操作属性和方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55
| Class clazz = null;
try { clazz = Class.forName("com.dream.xiaobo.reflect.impl.MyInterfaceImpl"); MyInterfaceImpl instance = (MyInterfaceImpl) clazz.newInstance(); Field id = clazz.getDeclaredField("id");
id.setAccessible(true); id.set(instance,1); System.out.println(instance.getId());
System.out.println("---------------"); Method method = clazz.getDeclaredMethod("student", String.class);
method.setAccessible(true);
method.invoke(instance,"xiaobo");
System.out.println("---------------");
Constructor constructor1 = clazz.getDeclaredConstructor(String.class); MyInterfaceImpl xiaobo = (MyInterfaceImpl)constructor1.newInstance("xiaobo");
System.out.println("---------------"); Constructor constructor2 = clazz.getDeclaredConstructor(Integer.class); constructor2.setAccessible(true); MyInterfaceImpl wyb = (MyInterfaceImpl)constructor2.newInstance(1);
}catch (ClassNotFoundException e){ e.printStackTrace(); } catch (NoSuchFieldException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } catch (InstantiationException e) { e.printStackTrace(); } catch (NoSuchMethodException e) { e.printStackTrace(); } catch (InvocationTargetException e) { e.printStackTrace(); }
|
越过泛型检查
1 2 3 4 5 6 7 8 9 10 11
| List<Integer> list = new ArrayList<>(); list.add(1); list.add(2);
Class clazz = list.getClass(); try { Method method = clazz.getMethod("add",Object.class);
method.invoke(list,"xiaobo");
System.out.println(list);
|
简单应用
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16
| public static void propertyUtils(Object type,String name,Object value){
Class<?> clazz = type.getClass();
try { Field field = clazz.getDeclaredField(name);
field.setAccessible(true); field.set(type,value);
} catch (NoSuchFieldException e) { e.printStackTrace(); } catch (IllegalAccessException e) { e.printStackTrace(); } }
|
1 2 3 4 5 6 7 8 9 10
| MyInterfaceImpl myInterface = new MyInterfaceImpl();
PropertyUtils.propertyUtils(myInterface,"id",1); PropertyUtils.propertyUtils(myInterface,"name","xiaobo"); System.out.println(myInterface.getId()); System.out.println(myInterface.getName());
Student student = new Student(); PropertyUtils.propertyUtils(student,"score",99.99); System.out.println(student.getScore());
|
正确的开始、微小的长进、然后持续、嘿、我是小博、带你一起看我目之所及的世界……